Lambda in A Nutshell
Background
Lambda can be classed as on demand code that does a specific function.
Let's simplify it!
Think of your computer at home, you will have a multiple of more powerful similar computers running within a data centre. A consumer can access the services on these computers via the Internet.
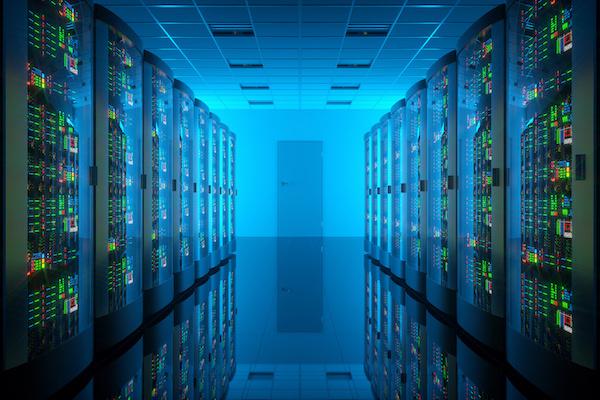
Abstration
Abstraction
What is abstraction? When you utilise your computer you don't have to keep changing the circuits or re-coding by replacing IC's or the processor logic via a low level language (Assembly language) while working on it. All you need to do is to access its keyboard, mouse and view it via the monitor. The programmes running within it (such as word, Excel etc.) provide you with a service to do your job. Hence we have a form of abstraction via peripherals when we access a home computer. We have access to the service on the computer but we do not need to understand the underlying complications of zeros and ones, switching circuits and the microprocessor. These are all hidden to the end user. Hence we can define this as a layer of abstraction.
Abstraction within Data-Centers
Within data centres such as AWS, the underlying physical computers are further abstracted by a virtualisation layer known as a Hypervisor.
The purpose of this is to pool all physical computing resources and present them to a consumer through abstraction. These new virtual resources can be consumed by end users as software resources without having to manage physical infrastructure. Some examples of this virtualisation layer are Xen, HyperV and VMware
Hypervisors
The result being multiple software based computers running on hypervisors that can be consumed by an end user. These are known as VMs or virtual machines. Operating systems such as Linux and Windows can be installed on these VMs.
Container Services
The computer industry came with another idea of further dissecting the Operating System running on these VMs into compartments to run specific functions or code. (Please note that container services can also run directly on hardware without a virtualisation layer or hypervisor).
You can think of the operating system within a VM or hardware computer as a house with a gate (entry point). The gate by which we access this resource through the Internet is its IP address mapped to a mac address within the OS, this is either hardcoded (Physical) or soft coded (Virtual) based on its use case.
Imagine apartments in London, You have a main entrance to the compound or block of flats via the main gate. The main compound can be further divided into blocks of flats with their own doors or gates (entrances).
The main compound being the OS and the containers being analogous to the apartments within the compound.
The gate of these containers is assigned specific IPs (internet addresses and port numbers) to access a specific service.
These container managements services are based on Docker Swarm, Google Kubernetes etc. (The images are hosted on docker containers within a compute node.
AWS have their own container management services known as ECS and EKS.
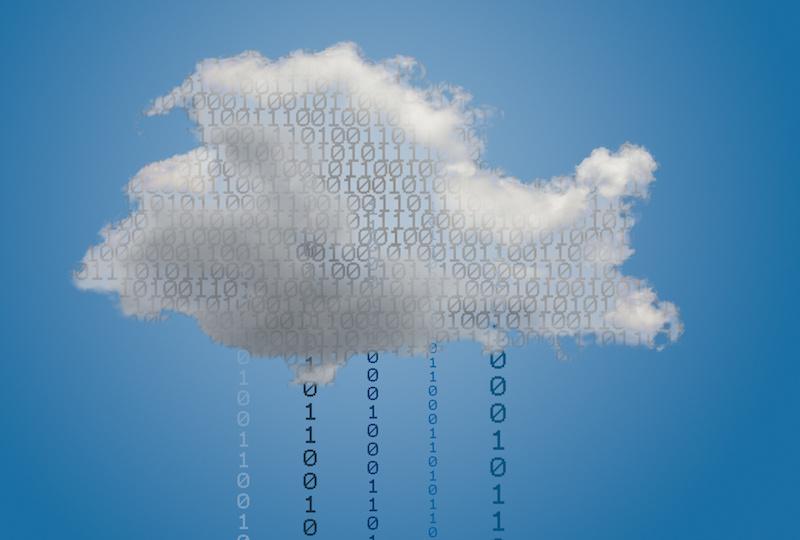
Lambda Defined
What is Lambda ?
Lambda is on demand code that runs on a managed AWS container service.
The on demand code will perform a function as a service and once it is executed the container that runs the service is terminated. Billed at 100ms intervals, Lambda can also grow based on demand through the deployment of more containers based on demand and performance. The service aspect of the container service is totally managed by AWS; hence all we have to do is deploy the code to consume it when and where necessary.
We have finally ended up with FaaS, which literally means function as a service, which is equivalent to Lambda on the AWS ecosystem.
Lambda components
Lambda is simple but can be a complex beast unless we understand the core concepts of lambda.
I will explain briefly the core concepts of Lambda. Once you understand these basic paradigms of lambda thoroughly, you will be able to deploy Lambdas with ease.
Objects attributes and methods
Before we delve into Lambda we need to understand what objects, attributes and methods are.
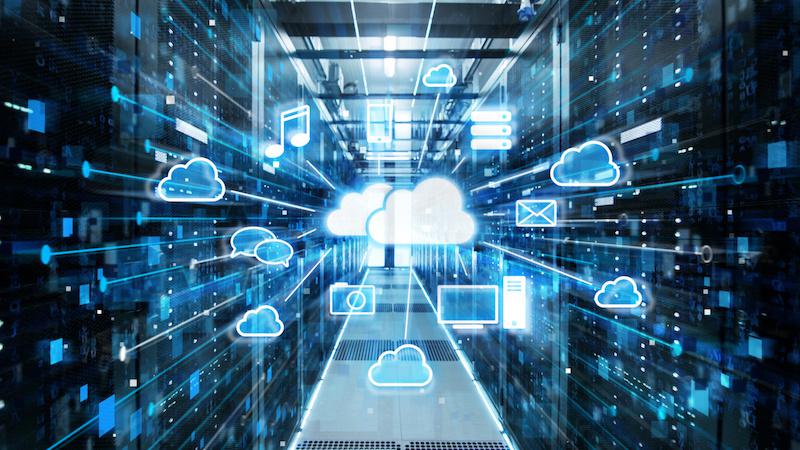
Object Oriented Mindset
Object
Think of your TV as an object. An object is tangible, you can feel it and and paly with it :). Similar to physical objects, in the computing world an object consists of logic that contains a series of on and off states "ones and zeros" that are tangible as electrical signals. Have you touched a live wire? It is tangible, but the voltages of the ones and zeros are too low to electrocute you :). If you had less resistance maybe it would be able to trigger some damage (based on Ohm's law). But we are safe here for the time being. Who knows if bodies are replaced by robotic material that may change the scenario a bit.
Attributes
What are attributes, attributes of my TV can be classified as:
If you were going to order a TV online you would need to go through its specification. The specification consists of its attributes.
Model: Samsung
Size: 42 inches
Screen: Plasma
Images: Colour
Operating Voltage: 120-230 vAC
Methods
Ok now I've bought my TV via amazon, what am I going to do with it?
Method1: Power On
Method2: Change Channel
Method3: Decrease Volume
Method4: Increase Volume
Method 5:Power Off
Hence if we write the following:
TV.Samsung.Power On, we end up with what you would define as the basis for object oriented programming.
It's another way of saying I need to watch the Simpsons so please switch on the TV. Although the syntax is not grammatical in English, it is a way of instructing a computer to do something via a high-level computer language. I can rig my computer to an IOT device and send the above command to the device to switch on my computer.
As you can see computing is not rocket science :)
What has object oriented programming (OOP) got to do with Lambda?
AWS Lambda supports Java, Go, PowerShell, Node.js, C#, Python, and Ruby. These are all object oriented programming languages, hence the reason for a grounding on object orientation.
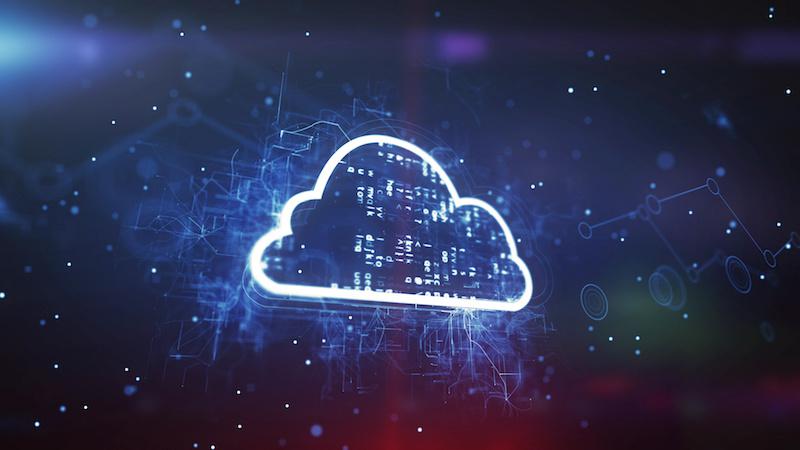
Lambda Objects
Crux of Lambda Context Object
A lambda function has what you would call a Context object.
Like our TV a lambda has its object and the following attributes.
There are more attributes than what are specified here but this is a high level introduction to grasp the core concepts of lambda in a logical way.
Context object properties (attributes)
Note: Most of these properties are generic, but the naming convention or code syntax can change based on the programming language (NodeJs/ Python etc.) that is utilized within the lambda to create the function. The following examples of properties are based on NodeJs. Our focus at the moment is to avoid being language specific, so that we could grasp the core concepts of lambda. We will look at more Context object properties for mobile apps (identity –) clientContext – (mobile apps) in due course.
Basically the context object defines properties of the lambda function itself.
The main properties (attributes) of the Context object are:
logGroupName: Name of the Log group that lambda logs to
logStreamName: Name of the log stream stream that lambda logs to
functionName: Name of the lambda function
memoryLimitInMB: Memory limit assigned to the lambda function by user
functionVersion: Version of the lambda function at runtime
invokeid: An ID assigned to the lambda function at invocation
awsRequestId: The identifier of the invocation request of the lambda function
invokedFunctionArn: The AWS resource name of the lambda function
Note: Logs are a way to keep track of events please refer to the links section at the bottom of this page for explanation of some of the following
Context object Method
getRemainingTimeInMillis: The remaining time of the function post execution, based on the time out assigned by the user when the lambda was configured.
This metric can be utilized to fine-tune your Lambda function duration settings.
Crux of Lambda Event Object
Most services on AWS can trigger an event that can execute a Lambda function. For example an object post via an API gateway can trigger a Lambda function. The event object passes the data to the lambda function via its Event object properties so that the function within the Lambda can manipulate a specific piece of data.
If we define an "event.a" in “object.attribute” format in object oriented programming) within the function. If the value of the attribute a = 5, the event object can pass the value of a=5 to the lambda function for manipulation at the runtime of the function.
Hence the event object can have multiple attributes that can be defined within the code of the function and utilized as placeholders to pass data to a lambda function for manipulation when an event is triggered.
For instance if I create a test event object with the following json values:
{
"a": "5.532",
"b": "10.235",
"op": "*"
}
We will be able get the result of the values of "a" & "b" based on the operand "op" via business logic defined in the code of the lambda function.
In this instance the result would be :
{
"a": 5.532,
"b": 10.235,
"op": "*",
"result": 56.62002
}
Crux of Lambda Handler function
Let's look at the following bit of NodeJs code within a lambda function
(Please ignore the "callback function until the next stage, this will be explained later).
exports.handler = function(event, context, callback)
NodeJs utilizes the export function to make available its code to an external program.
In this instance the handler function can be accessed by calling
filename.exportedfunctionname
If the node file was called "index.js" and the exportedfunctionname is defined as "handler" in this instance we can call the function externally utilising the following syntax.
index.handler
If we had another function called jondoe within the same index file and the same function is exported, we can access that function externally as shown below.
index.jondoe
If we created a new file called new.js and had the same function exported within the file as handler.
The function can now be called externally via:
new.handler
Similar syntax in Python:
If the name of the file is
hello.py
def lambda_handler(event, context):
The python function can be called externally via
hello.lambda_handler
It can get more complex with other languages, but if you understand the core the rest can be deduced by referring to AWS documentation and examples.
Crux of Lambda Callback Function {callback(x, y)}
Note this has been depreciated in NodeJs 8.10 (This only applies to NodeJs 6.10) (NodeJs 8.10 utilizes "return response")
Now we have a function that we can call and pass data to. What are we waiting for? Let's get some results shall we? It's time to rock and roll!
The lambda function has two call back parameter let us say they are x & y:
Before we Roll we need to understand what a parameter is!
A numerical or other measurable factor forming one of a set that defines a system or sets the conditions of its operation. (Courtesy of Wikipedia, Oops that's too complicated for me :)
Let's simplify it:
Parameter (x) (First parameter
(A)
If my lambda function fails due to the laws of nature not being met for some reason let's say that a parameter says something "error"("hey you are duped! you got your lambda wrong go back to school!") being the result of the function not functioning properly). This is known as “callback(error)”. Hence we can specify ("hey you are duped you got your lambda wrong go back to school!") within the call back function for the first parameter.
The NodeJs code will be as follows :
exports.handler = (event, context, callback) => {
// Scenario a
callback("hey you are duped you got your lambda wrong go back to school!");
};
The response would be
Response:
{
"errorMessage": "hey you are duped you got your lambda wrong go back to school!"
}
(B)
What if I don't define a callback parameter
The NodeJs code will be as follows :
exports.handler = (event, context, callback) => {
};
Response:
null
This means that the function is working properly and lambda initiates a NULL response to the user.
(C)
What if we add the following code by specifying a null value to the first parameter, as follows :
exports.handler = (event, context, callback) => {
// Scenario C
const response = {
statusCode: 200,
body: JSON.stringify('Hello from Lambda!'),
};
callback(null, response);
};
Response:
{
"statusCode": 200,
"body": "\"Hello from Lambda!\""
}
Based on some conditions that are met or not , we get three results via the first parameter, does it make sense? (Error, Null, Null and result, silence).
Parameter (y) in Scenario (C) the "Responce" object was the parameter (y)
(D)
What if I define the first callback parameter as null.
The NodeJs code will be as follows :
exports.handler = (event, context, callback) => {
// Scenario D
callback(null);
};
The response is still still Null as follows:
Response:
null
Ok to describe things as they are, finally strings or objects are returned to us by the function. (The laws of gravity may be changed but it is
as it is unless otherwise proven guilty in the court of lambda).
Callback (error) Means go and do your homework again (The function throws an error)
Callback() returns a Null value
Callback(Null, Returns) Returns the result
Callback(Null) Returns nothing to us although the function is successful
With the async await function that is based on promises, the syntax would be as follows (without the callback function)
console.log('Loading function');
exports.handler = async (event, context) => {
if (event.a === undefined || event.b === undefined || event.c === undefined) {
throw ("400 Invalid Input");
}
else if (typeof event.a !== 'number' || typeof event.a !== 'number' || typeof event.a !== 'number') {
throw ("Please enter numerical values for the event" + JSON.stringify(event));
}
else
var res = {};
res.a = Number(event.a);
res.b = Number(event.b);
res.c = Number(event.c);
res.d = res.a * res.b + res.c;
return res;
};
The response would be as follows if we don't input integer values through the test event.
Input=
{
"a": null,
"b": 10,
"c": 15
}
Response:
{
"errorMessage": "Please enter number for the event{\"a\":null,\"b\":10,
\"c\":15}"
}
If we enter integers via the trigger event the response would be as follows:
Test event values :
{
"a": 20,
"b": 10,
"c": 15
}
Response:
{
"a": 20,
"b": 10,
"c": 15,
"d": 215
}
If we input strings via the test event as follows :
{
"a": "20",
"b": "10',
"c": "15"
}
The response is as follows:
Response:
{
"errorMessage": "Please enter numericals values for the events{\"a\":\"20\",\"b\"
:\"10\",\"c\":\"15\"}"
}
CloudWatch logs will show what is logged as follows:
2019-01-19T14:40:39.258Z 4faefa19-ac87-4d68-8b78-59f4de42875b Loading function
14:40:39
START RequestId: 4faefa19-ac87-4d68-8b78-59f4de42875b Version: $LATEST
START RequestId: 4faefa19-ac87-4d68-8b78-59f4de42875b Version: $LATEST
14:40:39
2019-01-19T14:40:39.262Z 4faefa19-ac87-4d68-8b78-59f4de42875b {"errorMessage":"Please enter numericals for the events{\"a\":\"20\",\"b\
":\"10\",\"c\":\"15\"}"}
2019-01-19T14:40:39.262Z 4faefa19-ac87-4d68-8b78-59f4de42875b
{
"errorMessage": "Please enter numericals for the events{\"a\":\"20\",\"b\
":\"10\",\"c\":\"15\"}"
}
14:40:39
END RequestId: 4faefa19-ac87-4d68-8b78-59f4de42875b
END RequestId: 4faefa19-ac87-4d68-8b78-59f4de42875b
14:40:39
REPORT RequestId: 4faefa19-ac87-4d68-8b78-59f4de42875b Duration: 5.25 ms Billed Duration: 100 ms Memory Size: 128 MB Max Memory Used: 19 MB
REPORT RequestId: 4faefa19-ac87-4d68-8b78-59f4de42875b Duration: 5.25 ms Billed Duration: 100 ms Memory Size: 128 MB Max Memory Used: 19 MB
Lambda@Edge
This is an integration between CloudFront CDN mentioned earlier and Lambda.
In this instance Lambda is able to transform requests and responses between the viewer and Origin server, If Cloud front is being utilised as a CDN.
Lambda deployment Methods
Deploy via CLI (AWS CLI needs to be installed on your computer and the computer needs to have programmatic access to the AWS account.
Zip (Upload Zip (code) file via AWS console)
Author From Scratch (Write your function via the AWS console code editor)
Blue Prints (Deploy from existing blueprints within the AWS ECOsystem)
Serverless Framework (Deploy function vi the serverless framework from your computer or a deployment computer and a CI/CD pipeline)
AWS Serverless Application repository (The application repository functions or services can be called via the AWS console to deploy the function and related service via CloudFormation) Similarly the application can be deleted via Cloud Formation.
Lambda IAM Roles
In AWS none of the services have access to each other or resources unless granted via IAM roles and policies. Only the root account can grant such permissions via I am users (programmatic access or logon access) and roles. The permissions are granted via policies. It is a best practice to create groups and roles to grant privileges within AWS.
Lambda privileges
As mentioned above the syntax of a json policy to give a lambda function privileges to another service would be as follows. In this instance access to cloudwatch logs.
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"logs:CreateLogGroup",
"logs:CreateLogStream",
"logs:PutLogEvents"
],
"Resource": "arn:aws:logs:*:*:*"
}
]
}
The above policy says, hey I am going to give this particular lambda function privileges to create a log group, create logs streams and log events to those streams.
We will delve into these policies later, they are easy to understand once the basics are.
Effect (Allow or deny an action)
Action ( what policy will allow you to do)
Resource (Amazon resource name of the service that we are going to perform actions on a "subject".
The policy is assigned via an IAM role in AWS.
Lambda logging (CloudWatch Logs)
Amazon CloudWatch Logs can be utilized to monitor, store, and access your log files for AWS lambda.
First of all we need to give the function access to a cloud watch Log Group and Log Stream via an IAM role and a policy(jason document).
So let's have some fun with Lambda now! And build some more functioning lambdas.
Lambda Versioning
These values can be called through AWS CLI (AWS command line)
aws lambda get-function --function-name "test"
Which would list the following:
aws lambda get-function --function-name test
{
"Configuration": {
"FunctionName": "test",
"FunctionArn": "arn:aws:lambda:eu-west-2:aws-account-number:function:test",
"Runtime": "nodejs8.10",
"Role": "arn:aws:iam::aws-account-number:role/lambda
_basic_execution",
"Handler": "index.handler",
"CodeSize": 1291,
"Description": "",
"Timeout": 3,
"MemorySize": 128,
"LastModified": "2019-01-19T16:59:21.374+0000",
"CodeSha256": "yuwXBqZLe9K3L/e4P
CrBN474nZG+Iax6Zz7ypV95Ahc=",
"Version": "$LATEST",
"TracingConfig": {
"Mode": "PassThrough"
}
},
"Code": {
"RepositoryType": "S3",
"Location""https://awslambda-eu-west-2-tasks.s3.eu-west-2.amazonaws.com*
By default when we create a function the versioning process creates an alias called $latest. This is basically a pointer to the latest update of our code and the function properties, when we edited the function and saved it. (This would be like the development version of our function)
We can further publish the function and the process adds a version number such as version 1 for example to show that the function is production ready.
Note: Code and handler editing is only available for the $LATEST version.
Once published the published version code and the handler cannot be edited.
Once published the function gets an Identifier, this published function can be called via its identifier : 1 being the identifier of the published function.
aws lambda get-function --function-name test:1
{
"Configuration": {
"FunctionName": "test",
"FunctionArn": "arn:aws:lambda:eu-west-2:aws-account-number:function:test:1",
"Runtime": "nodejs8.10",
"Role": "arn:aws:iam::aws-account-number:role/lambda_basi
c_execution",
"Handler": "index.handler",
"CodeSize": 1291,
"Description": "Version 1",
"Timeout": 3,
"MemorySize": 128,
"LastModified": "2019-01-19T16:59:21.374+0000",
"CodeSha256": "yuwXBqZLe9K3L/e4P
CrBN474nZG+Iax6Zz7ypV95
Ahc=",
"Version": "1",
"TracingConfig": {
"Mode": "PassThrough"
}
},
"Code": {
"RepositoryType": "S3",
"Location": "https://awslambda-eu-west-2-tasks.s3.eu-west-2.amazonaws.com*
We can further create an alias for this function and it can be called via its alias.
aws lambda get-function --function-name test --qualifier "alias"
Note: Aliases are also called qualifiers, the default being "$LATEST" if the function isn't published. "$LATEST" being the latest version of the uploaded code .
AWS CLI commands can be used to upload, update, publish a function and also change the handler of the function based on the code.
aws lambda update-function-code --zip-file=fileb://xxx.zip --function-name
test
aws lambda update-function-code --zip-file=fileb://yyy.zip --function-name test
aws lambda update-function-configuration --function-name test --handler xxx.handler
aws lambda update-function-code --zip-file=fileb://xxx.zip --function-name test --publish
Note: The Amazon resource name gets updated with the version number (identifier) as shown below (Highlighted in red).
FunctionArn": "arn:aws:lambda:eu-west-2:aws-account-number:function:test:2",
Timeouts
A timeout for a lambda function can be specified in minutes and seconds in case of a loop, a wait condition or faulty code. After setting an initial value for the time out based on assumption, it would be best to set this value based on metrics returned by cloud watch logs. Please note that the default billing unit is 100ms So even if your function is executed and finished within 20ms you will still be billed by a 100 ms billing cycle.
Allocated Memory
The user defined memory for the function also has an impact on the CPU units allocated to the function. A range from 128MB to 3008MB can be assigned to the lambda function. (Please note that the capital M stands for bytes and not bits).
Environment Variables
Multiple Environment variables can be provided to a lambda function via the environment variable option. Either through the console or AWS CLI.
Example:
(update-function-configuration --function-name --environment)